Python基础(二十三):面向对象之继承介绍
面向对象之继承介绍
一、继承的概念
生活中的继承,⼀般指的是子女继承父辈的财产。
- 拓展1:经典类或旧式类
不由任意内置类型派生出的类,称之为经典类。
class 类名:
代码
......
- 拓展2:新式类
class 类名(object):
代码
Python面向对象的继承指的是多个类之间的所属关系,即子类默认继承父类的所有属性和方法,具体如下:
# ⽗类A
class A(object):
def __init__(self):
self.num = 1
def info_print(self):
print(self.num)
# ⼦类B
class B(A):
pass
result = B()
result.info_print() # 1
在Python中,所有类默认继承object类,object类是顶级类或基类;其他⼦类叫做派生类。
二、单继承
故事主线:⼀个煎饼果子老师傅,在煎饼果子界摸爬滚打多年,研发了⼀套精湛的摊煎饼果子的技术。师父要把这套技术传授给他的唯⼀的最得意的徒弟。
分析:徒弟是不是要继承师父的所有技术?
# 1. 师⽗类
class Master(object):
def __init__(self):
self.kongfu = '[古法煎饼果⼦配⽅]'
def make_cake(self):
print(f'运⽤{self.kongfu}制作煎饼果⼦')
# 2. 徒弟类
class Prentice(Master):
pass
# 3. 创建对象daqiu
daqiu = Prentice()
# 4. 对象访问实例属性
print(daqiu.kongfu)
# 5. 对象调⽤实例⽅法
daqiu.make_cake()
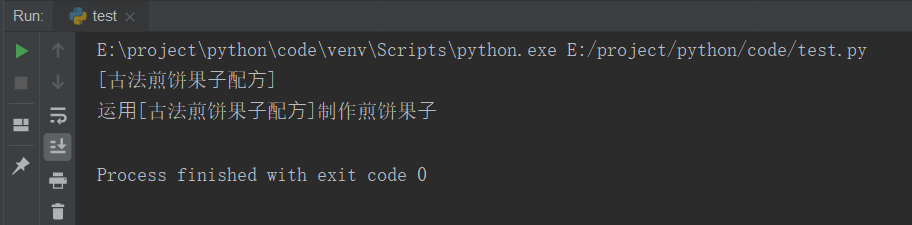
三、多继承
故事推进:daqiu是个爱学习的好孩子,想学习更多的煎饼果子技术,于是,在百度搜索到CSDN的博主报班学习煎饼果子技术。
所谓多继承意思就是⼀个类同时继承了多个父类。
class Master(object):
def __init__(self):
self.kongfu = '[古法煎饼果⼦配⽅]'
def make_cake(self):
print(f'运⽤{self.kongfu}制作煎饼果⼦')
# 创建学校类
class School(object):
def __init__(self):
self.kongfu = '[Lansonli煎饼果⼦配⽅]'
def make_cake(self):
print(f'运⽤{self.kongfu}制作煎饼果⼦')
class Prentice(School, Master):
pass
daqiu = Prentice()
print(daqiu.kongfu)
daqiu.make_cake()
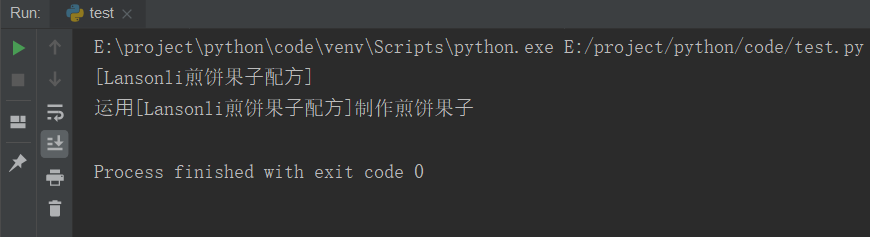
注意:当⼀个类有多个父类的时候,默认使用第⼀个父类的同名属性和方法。
四、子类重写父类同名方法和属性
故事:daqiu掌握了师父和培训的技术后,自己潜心钻研出自己的独门配方的⼀套全新的煎饼果子技术。
class Master(object):
def __init__(self):
self.kongfu = '[古法煎饼果⼦配⽅]'
def make_cake(self):
print(f'运⽤{self.kongfu}制作煎饼果⼦')
class School(object):
def __init__(self):
self.kongfu = '[Lansonli煎饼果⼦配⽅]'
def make_cake(self):
print(f'运⽤{self.kongfu}制作煎饼果⼦')
# 独创配⽅
class Prentice(School, Master):
def __init__(self):
self.kongfu = '[独创煎饼果⼦配⽅]'
def make_cake(self):
print(f'运⽤{self.kongfu}制作煎饼果⼦')
daqiu = Prentice()
print(daqiu.kongfu)
daqiu.make_cake()
print(Prentice.__mro__)
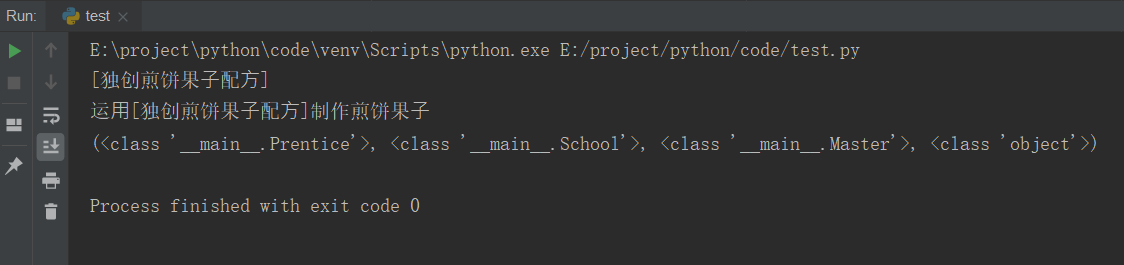
子类和父类具有同名属性和方法,默认使用子类的同名属性和方法。
五、子类调用父类的同名方法和属性
故事:很多顾客都希望也能吃到古法和Lansonli的技术的煎饼果子。
class Master(object):
def __init__(self):
self.kongfu = '[古法煎饼果⼦配⽅]'
def make_cake(self):
print(f'运⽤{self.kongfu}制作煎饼果⼦')
class School(object):
def __init__(self):
self.kongfu = '[Lansonli煎饼果⼦配⽅]'
def make_cake(self):
print(f'运⽤{self.kongfu}制作煎饼果⼦')
class Prentice(School, Master):
def __init__(self):
self.kongfu = '[独创煎饼果⼦配⽅]'
def make_cake(self):
# 如果是先调⽤了⽗类的属性和⽅法,⽗类属性会覆盖⼦类属性,故在调⽤属性前,先调⽤⾃⼰⼦类的初始化
self.__init__()
print(f'运⽤{self.kongfu}制作煎饼果⼦')
# 调⽤⽗类⽅法,但是为保证调⽤到的也是⽗类的属性,必须在调⽤⽅法前调⽤⽗类的初始化
def make_master_cake(self):
Master.__init__(self)
Master.make_cake(self)
def make_school_cake(self):
School.__init__(self)
School.make_cake(self)
daqiu = Prentice()
daqiu.make_cake()
daqiu.make_master_cake()
daqiu.make_school_cake()
daqiu.make_cake()
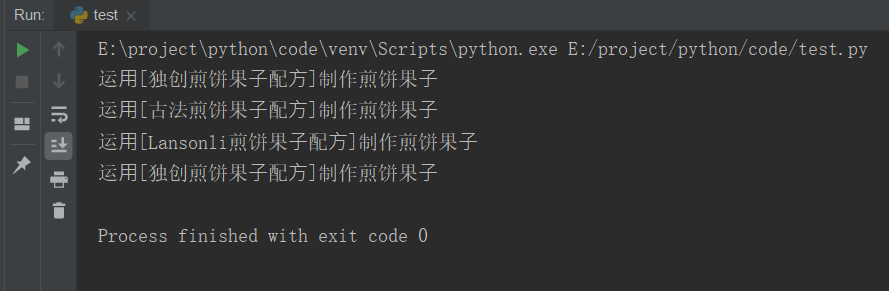
六、多层继承
故事:N年后,daqiu老了,想要把所有技术传承给自己的徒弟。
class Master(object):
def __init__(self):
self.kongfu = '[古法煎饼果⼦配⽅]'
def make_cake(self):
print(f'运⽤{self.kongfu}制作煎饼果⼦')
class School(object):
def __init__(self):
self.kongfu = '[Lansonli煎饼果⼦配⽅]'
def make_cake(self):
print(f'运⽤{self.kongfu}制作煎饼果⼦')
class Prentice(School, Master):
def __init__(self):
self.kongfu = '[独创煎饼果⼦配⽅]'
def make_cake(self):
self.__init__()
print(f'运⽤{self.kongfu}制作煎饼果⼦')
def make_master_cake(self):
Master.__init__(self)
Master.make_cake(self)
def make_school_cake(self):
School.__init__(self)
School.make_cake(self)
# 徒孙类
class Tusun(Prentice):
pass
xiaoqiu = Tusun()
xiaoqiu.make_cake()
xiaoqiu.make_school_cake()
xiaoqiu.make_master_cake()
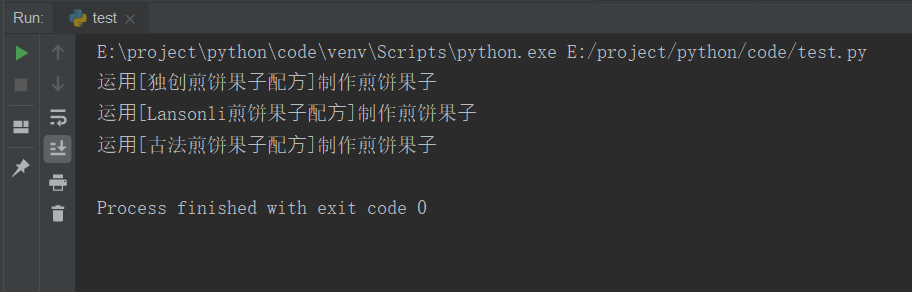
七、super()调用父类方法
class Master(object):
def __init__(self):
self.kongfu = '[古法煎饼果⼦配⽅]'
def make_cake(self):
print(f'运⽤{self.kongfu}制作煎饼果⼦')
class School(Master):
def __init__(self):
self.kongfu = '[Lansonli煎饼果⼦配⽅]'
def make_cake(self):
print(f'运⽤{self.kongfu}制作煎饼果⼦')
# ⽅法2.1
# super(School, self).__init__()
# super(School, self).make_cake()
# ⽅法2.2
super().__init__()
super().make_cake()
class Prentice(School):
def __init__(self):
self.kongfu = '[独创煎饼果⼦技术]'
def make_cake(self):
self.__init__()
print(f'运⽤{self.kongfu}制作煎饼果⼦')
# ⼦类调⽤⽗类的同名⽅法和属性:把⽗类的同名属性和⽅法再次封装
def make_master_cake(self):
Master.__init__(self)
Master.make_cake(self)
def make_school_cake(self):
School.__init__(self)
School.make_cake(self)
# ⼀次性调⽤⽗类的同名属性和⽅法
def make_old_cake(self):
# ⽅法⼀:代码冗余;⽗类类名如果变化,这⾥代码需要频繁修改
# Master.__init__(self)
# Master.make_cake(self)
# School.__init__(self)
# School.make_cake(self)
# ⽅法⼆: super()
# ⽅法2.1 super(当前类名, self).函数()
# super(Prentice, self).__init__()
# super(Prentice, self).make_cake()
# ⽅法2.2 super().函数()
super().__init__()
super().make_cake()
daqiu = Prentice()
daqiu.make_old_cake()
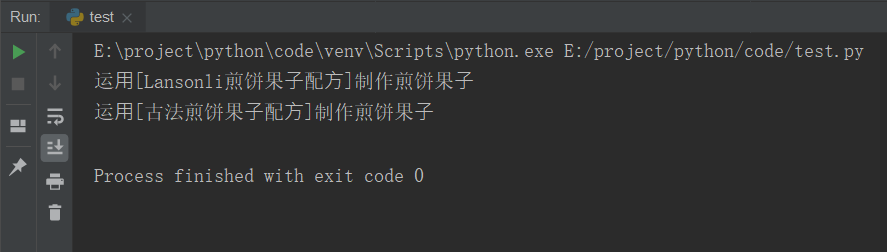
注意:使用super() 可以自动查找父类。调用顺序遵循 __mro__ 类属性的顺序。比较适合单继承使用。
八、私有权限
1、定义私有属性和方法
在Python中,可以为实例属性和方法设置私有权限,即设置某个实例属性或实例方法不继承给子类。
故事:daqiu把技术传承给徒弟的同时,不想把自己的钱(20个亿)继承给徒弟,这个时候就要为钱这个实例属性设置私有权限。
设置私有权限的方法:在属性名和方法名前面加上两个下划线 __。
class Master(object):
def __init__(self):
self.kongfu = '[古法煎饼果⼦配⽅]'
def make_cake(self):
print(f'运⽤{self.kongfu}制作煎饼果⼦')
class School(object):
def __init__(self):
self.kongfu = '[Lansonli煎饼果⼦配⽅]'
def make_cake(self):
print(f'运⽤{self.kongfu}制作煎饼果⼦')
class Prentice(School, Master):
def __init__(self):
self.kongfu = '[独创煎饼果⼦配⽅]'
# 定义私有属性
self.__money = 20
# 定义私有⽅法
def __info_print(self):
print(self.kongfu)
print(self.__money)
def make_cake(self):
self.__init__()
print(f'运⽤{self.kongfu}制作煎饼果⼦')
def make_master_cake(self):
Master.__init__(self)
Master.make_cake(self)
def make_school_cake(self):
School.__init__(self)
School.make_cake(self)
# 徒孙类
class Tusun(Prentice):
pass
daqiu = Prentice()
# 对象不能访问私有属性和私有⽅法
# print(daqiu.__money)
# daqiu.__info_print()
xiaoqiu = Tusun()
# ⼦类⽆法继承⽗类的私有属性和私有⽅法
# print(xiaoqiu.__money) # ⽆法访问实例属性__money
# xiaoqiu.__info_print()
注意:私有属性和私有方法只能在类里面访问和修改。
2、获取和修改私有属性值
在Python中,⼀般定义函数名 get_xx 用来获取私有属性,定义 set_xx 用来修改私有属性值。
class Master(object):
def __init__(self):
self.kongfu = '[古法煎饼果⼦配⽅]'
def make_cake(self):
print(f'运⽤{self.kongfu}制作煎饼果⼦')
class School(object):
def __init__(self):
self.kongfu = '[Lansonli煎饼果⼦配⽅]'
def make_cake(self):
print(f'运⽤{self.kongfu}制作煎饼果⼦')
class Prentice(School, Master):
def __init__(self):
self.kongfu = '[独创煎饼果⼦配⽅]'
self.__money = 20
# 获取私有属性
def get_money(self):
return self.__money
# 修改私有属性
def set_money(self):
self.__money = 500
def __info_print(self):
print(self.kongfu)
print(self.__money)
def make_cake(self):
self.__init__()
print(f'运⽤{self.kongfu}制作煎饼果⼦')
def make_master_cake(self):
Master.__init__(self)
Master.make_cake(self)
def make_school_cake(self):
School.__init__(self)
School.make_cake(self)
# 徒孙类
class Tusun(Prentice):
pass
daqiu = Prentice()
xiaoqiu = Tusun()
# 调⽤get_money函数获取私有属性money的值
print(xiaoqiu.get_money())
# 调⽤set_money函数修改私有属性money的值
xiaoqiu.set_money()
print(xiaoqiu.get_money())
- 点赞
- 收藏
- 关注作者
评论(0)