1小时学会通过Java Swing Design设计java图形化
编辑
环境与工具:
系统环境:win10
开发工具:Eclipse IDE for Java Developers - 2020-09
数据库:MySQL5.6
学习目标:
一般大一结束的时候需要做一个类似一下内容的javaswing项目,故而咱们的学习目标如下:

其中设计到的控件:文本框、输入框、按钮、表格,数据库是JDBC绑定数据集<List>类型就行。
目录
1、项目创建
使用eclipse直接创建一个java的空项目即可,唯一要注意的就是创建【java】的时候不是点击【class】而是创建【JFrame】,通过【JFrame】才能使用设计工具【Design】。
编辑
这里点【other】因为【JFrame】在这个选项栏中。



编辑

编辑2、绝对位置布局Absolute layout
使用这个方式方便拖拽,很方便:
编辑
编辑

编辑3、工具栏简述:
编辑
编辑
编辑
编辑
编辑

编辑4、常用控件简述
1、顶层容器:JFrame(窗体),JDialog(对话窗)
2、中间容器:JPanel(面板),(滚动面板)JScrollPane,(分割面板)JSplitPane,(工具栏)JToolBar
3、菜单栏:JMenuBar
4、基本组件:
英文名 | 对照中文 |
JLabel | 标签 |
JButton | 按钮 |
JTextArea | 文本区 |
JTextField | 文本框 |
JRadioButton | 单选按钮 |
JCheckBox | 复选框 |
JPasswordField | 密码框 |
JComboBox | 下拉列表框 |
JList | 列表 |
JTable | 表格 |
画的有些乱,但是基本上一眼就能看出来是啥:
编辑
表格JTable:
JPanel设置上下布局,JScrollPane设置,这样才能显示table的标题列
编辑
添加一些测试数据:
编辑
效果:
编辑
5、JDBC
需要数据库jar包
mysql-connector-java-5.1.7bin.jar
下载链接:https://download.csdn.net/download/feng8403000/85610502
MySQL
DROP TABLE IF EXISTS `users`;
CREATE TABLE `users` (
`id` int(8) NOT NULL AUTO_INCREMENT,
`createDate` datetime(0) NOT NULL,
`userName` varchar(50) CHARACTER SET utf8 COLLATE utf8_general_ci NOT NULL,
`sex` varchar(2) CHARACTER SET utf8 COLLATE utf8_general_ci NOT NULL,
`introduce` varchar(255) CHARACTER SET utf8 COLLATE utf8_general_ci NOT NULL,
PRIMARY KEY (`id`) USING BTREE
) ENGINE = InnoDB AUTO_INCREMENT = 3 CHARACTER SET = utf8 COLLATE = utf8_general_ci ROW_FORMAT = Compact;
-- ----------------------------
-- Records of users
-- ----------------------------
INSERT INTO `users` VALUES (1, '2022-06-11 12:55:02', '王语嫣', '女', '琅嬛福地,神仙姐姐。');
INSERT INTO `users` VALUES (2, '2022-06-11 12:56:05', '小龙女', '女', '冰山美人');
SET FOREIGN_KEY_CHECKS = 1;

JDCB_Demo
package com.item.jdbc;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
/**
* 数据库工厂
* @author Administrator
*
*/
public class FactoryDB {
/**
* 驱动位置
*/
private static final String driver ="com.mysql.jdbc.Driver";
/**
* 数据库链接路径·必背
*/
private static final String url ="jdbc:mysql://127.0.0.1:3306/mytest?characterEncoding=utf-8";
/**
* 数据库账号
*/
private static final String user ="root";
/**
* 数据库密码
*/
private static final String pwd ="12345678";
/**
* 静态块引入数据库驱动·解决包位置问题
*/
static {
try {
Class.forName(driver);
} catch (ClassNotFoundException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
/**
* 数据库链接
* @return
*/
public static Connection getConn() {
Connection conn = null;
try {
conn = DriverManager.getConnection(url,user,pwd);
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return conn;
}
/**
* 关闭数据库连接
* @param conn
* @param pr
* @param re
*/
public static void close(Connection conn,PreparedStatement pr, ResultSet re) {
try {
if (re!=null) {
re.close();
}
if(pr!=null){
pr.close();
}
if(conn!=null){
conn.close();
}
} catch (Exception e) {
e.printStackTrace();
}
}
}

封装模型:
package com.item.model;
import java.util.Date;
public class Users {
@Override
public String toString() {
return "Users [id=" + id + ", creaetDate=" + creaetDate + ", userName=" + userName + ", sex=" + sex
+ ", introduce=" + introduce + "]";
}
private int id;
private Date creaetDate;
private String userName;
private String sex;
private String introduce;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public Date getCreaetDate() {
return creaetDate;
}
public void setCreaetDate(Date creaetDate) {
this.creaetDate = creaetDate;
}
public String getUserName() {
return userName;
}
public void setUserName(String userName) {
this.userName = userName;
}
public String getSex() {
return sex;
}
public void setSex(String sex) {
this.sex = sex;
}
public String getIntroduce() {
return introduce;
}
public void setIntroduce(String introduce) {
this.introduce = introduce;
}
}

DAO层数据:
package com.item.dao;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import com.item.jdbc.FactoryDB;
import com.item.model.Users;
public class UsersDAO {
/**
* 查询所有
* @return
*/
public static ArrayList<Users> GetInfo(String userName){
Connection conn = FactoryDB.getConn();
String sql=null;
if(userName==null) {
sql="select * from users";
}else {
sql="select * from users where userName like '%"+userName+"%'";
}
ArrayList<Users> list = new ArrayList<Users>();
try {
PreparedStatement pr = conn.prepareStatement(sql);
ResultSet re = pr.executeQuery();
while (re.next()) {
Users u = new Users();
u.setId(re.getInt(1));
u.setCreaetDate(re.getDate(2));
u.setUserName(re.getString(3));
u.setSex(re.getString(4));
u.setIntroduce(re.getString(5));
list.add(u);
}
FactoryDB.close(conn, pr, re);
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return list;
}
/**
* 添加
* @param userName
* @param sex
* @param introduce
* @return
*/
public static boolean AddInfo(String userName,String sex,String introduce) {
Connection conn = FactoryDB.getConn();
String sql=String.format("insert into users values(0,now(),'%s','%s','%s')", userName,sex,introduce);
try {
PreparedStatement pr = conn.prepareStatement(sql);
int rows = pr.executeUpdate();
FactoryDB.close(conn, pr, null);
return rows>0;
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return false;
}
/**
* 修改
* @param id
* @param introduce
* @return
*/
public static boolean UpdateById(int id,String introduce) {
Connection conn = FactoryDB.getConn();
String sql=String.format("update users set introduce='%s' where id=%d", introduce,id);
try {
PreparedStatement pr = conn.prepareStatement(sql);
int rows = pr.executeUpdate();
FactoryDB.close(conn, pr, null);
return rows>0;
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return false;
}
/**
* 删除
* @param id
* @return
*/
public static boolean DeleteById(int id) {
Connection conn = FactoryDB.getConn();
String sql=String.format("delete from users where id=%d", id);
try {
PreparedStatement pr = conn.prepareStatement(sql);
int rows = pr.executeUpdate();
FactoryDB.close(conn, pr, null);
return rows>0;
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return false;
}
}

UI层添加数据:
JTable需要绑定一下俩数据:
//添加标题
Vector vTitle = new Vector();
//添加数据
Vector vdate = new Vector();
//绑定到控件
table.setModel(new DefaultTableModel(vdate,vTitle));

package com.item.ui;
import java.awt.BorderLayout;
import java.awt.EventQueue;
import java.util.ArrayList;
import java.util.Vector;
import javax.swing.JFrame;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.border.EmptyBorder;
import javax.swing.JScrollPane;
import javax.swing.JButton;
import javax.swing.JTextField;
import javax.swing.JTable;
import javax.swing.table.DefaultTableModel;
import com.item.dao.UsersDAO;
import com.item.model.Users;
import java.awt.event.ActionListener;
import java.awt.event.ActionEvent;
public class utest extends JFrame {
/**
* 序列
*/
private static final long serialVersionUID = 1L;
private JPanel contentPane;
private JTextField userName;
private JTable table;
/**
* Launch the application.
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
utest frame = new utest();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
/**
* Create the frame.
*/
public utest() {
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 100, 667, 598);
contentPane = new JPanel();
contentPane.setBorder(new EmptyBorder(5, 5, 5, 5));
contentPane.setLayout(new BorderLayout(0, 0));
setContentPane(contentPane);
JPanel panel = new JPanel();
contentPane.add(panel, BorderLayout.NORTH);
//添加按钮
JButton btnNewButton = new JButton("\u6DFB\u52A0");
btnNewButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
AddUsers users = new AddUsers();
users.setVisible(true);
}
});
panel.add(btnNewButton);
userName = new JTextField();
panel.add(userName);
userName.setColumns(20);
//查询按钮
JButton btnNewButton_2 = new JButton("\u67E5\u8BE2");
btnNewButton_2.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
bind(userName.getText());
}
});
panel.add(btnNewButton_2);
JPanel panel_1 = new JPanel();
contentPane.add(panel_1, BorderLayout.SOUTH);
//删除
JButton btnNewButton_1 = new JButton("\u9009\u4E2D\u884C\u70B9\u8FD9\u91CC\u5220\u9664");
btnNewButton_1.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
int i = table.getSelectedRow();
if (i==-1) {
JOptionPane.showMessageDialog(null,"请选择删除的行");
return ;
}
String id = table.getValueAt(i, 0).toString();
boolean isf = UsersDAO.DeleteById(Integer.parseInt(id));
if (isf) {
JOptionPane.showMessageDialog(null, "删除成功!!!");
} else {
JOptionPane.showMessageDialog(null, "删除失败!!!");
}
bind(null);
}
});
panel_1.add(btnNewButton_1);
//刷新按钮
JButton btnNewButton_3 = new JButton("\u6570\u636E\u5237\u65B0");
btnNewButton_3.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
bind(null);
}
});
panel_1.add(btnNewButton_3);
JScrollPane scrollPane = new JScrollPane();
contentPane.add(scrollPane, BorderLayout.CENTER);
table = new JTable();
scrollPane.setViewportView(table);
bind(null);
}
//加载数据
public void bind(String userName) {
//添加标题
Vector<String> vTitle = new Vector<String>();
vTitle.add("编号");
vTitle.add("创建时间");
vTitle.add("用户名");
vTitle.add("性别");
vTitle.add("简介");
//添加数据
Vector vdate = new Vector<Users>();
ArrayList<Users> list = UsersDAO.GetInfo(userName);
for (Users u : list) {
Vector v = new Vector();
v.add(u.getId());
v.add(u.getCreaetDate());
v.add(u.getUserName());
v.add(u.getSex());
v.add(u.getIntroduce());
vdate.add(v);
}
table.setModel(new DefaultTableModel(vdate,vTitle));
}
}

效果:
编辑
添加:如果有单选按钮得分组
编辑
为了方便操作,别忘改一个控件的名称:
编辑

双击添加按钮:

编辑
添加编码:
package com.item.ui;
import java.awt.BorderLayout;
import java.awt.EventQueue;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.border.EmptyBorder;
import com.item.dao.UsersDAO;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JRadioButton;
import javax.swing.SwingConstants;
import javax.swing.JTextField;
import javax.swing.JTextArea;
import javax.swing.JButton;
import javax.swing.ButtonGroup;
import java.awt.event.ActionListener;
import java.awt.event.ActionEvent;
public class AddUsers extends JFrame {
private JPanel contentPane;
private JTextField userName;
private final ButtonGroup buttonGroup = new ButtonGroup();
public utest utest;
/**
* Launch the application.
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
AddUsers frame = new AddUsers();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
/**
* Create the frame.
*/
public AddUsers() {
setBounds(100, 100, 589, 457);
contentPane = new JPanel();
contentPane.setBorder(new EmptyBorder(5, 5, 5, 5));
setContentPane(contentPane);
contentPane.setLayout(null);
JLabel lblNewLabel = new JLabel("\u7528\u6237\u540D");
lblNewLabel.setBounds(100, 88, 76, 29);
contentPane.add(lblNewLabel);
JLabel lblNewLabel_1 = new JLabel("\u7B80\u4ECB");
lblNewLabel_1.setBounds(100, 209, 54, 15);
contentPane.add(lblNewLabel_1);
JRadioButton sex1 = new JRadioButton("\u7537");
buttonGroup.add(sex1);
sex1.setSelected(true);
sex1.setBounds(200, 141, 121, 23);
contentPane.add(sex1);
JLabel lblNewLabel_2 = new JLabel("\u6027\u522B");
lblNewLabel_2.setBounds(100, 145, 54, 15);
contentPane.add(lblNewLabel_2);
JRadioButton sex2 = new JRadioButton("\u5973");
buttonGroup.add(sex2);
sex2.setBounds(357, 141, 121, 23);
contentPane.add(sex2);
userName = new JTextField();
userName.setBounds(160, 92, 278, 21);
contentPane.add(userName);
userName.setColumns(10);
JTextArea introduce = new JTextArea();
introduce.setBounds(164, 204, 291, 117);
contentPane.add(introduce);
JButton btnNewButton = new JButton("\u6DFB\u52A0");
btnNewButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String name = userName.getText();
boolean isf = sex1.isSelected();
String sex=isf?"男":"女";
String intro = introduce.getText();
boolean addInfo = UsersDAO.AddInfo(name, sex, intro);
JOptionPane.showMessageDialog(null, addInfo?"添加成功!!!":"添加失败!!!");
}
});
btnNewButton.setBounds(231, 357, 93, 23);
contentPane.add(btnNewButton);
}
}

增删查演示完毕。
修改就是删除的获取数据跟添加组合在一起就行了。
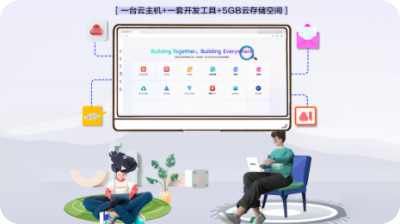
华为开发者空间发布
让每位开发者拥有一台云主机
- 点赞
- 收藏
- 关注作者
评论(0)