Python 中的3Dplot
➤01 3D plot
1.基本语法
在安装matplotlib之后,自动安装有 mpl_toolkits.mplot3d。
#Importing Libraries
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import axes3d
#3D Plotting
fig = plt.figure()
ax = plt.axes(projection="3d")
#Labeling
ax.set_xlabel('X Axes')
ax.set_ylabel('Y Axes')
ax.set_zlabel('Z Axes')
plt.show()
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
2.Python Cmd
使用pythoncmd 插入相应的语句。
3.举例
(1) Ex1
#!/usr/local/bin/python
# -*- coding: gbk -*-
#============================================================
# TEST2.PY -- by Dr. ZhuoQing 2020-11-16
#
# Note:
#============================================================
from headm import *
from mpl_toolkits.mplot3d import axes3d
ax = plt.axes(projection='3d')
x = [1,2,3,4,5,6,7,8,9]
y = [2,3,4,6,7,8,9,5,1]
z = [5,6,2,4,8,6,5,6,1]
ax.plot3D(x,y,z)
ax.set_xlabel('X Axes')
ax.set_ylabel('Y Axes')
ax.set_zlabel('Z Axes')
plt.show()
#------------------------------------------------------------
# END OF FILE : TEST2.PY
#============================================================
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
▲ 3D plot的演示
(2) Ex2
from mpl_toolkits.mplot3d import axes3d
ax = plt.axes(projection='3d')
angle = linspace(0, 2*pi*5, 400)
x = cos(angle)
y = sin(angle)
z = linspace(0, 5, 400)
ax.plot3D(x,y,z)
ax.set_xlabel('X Axes')
ax.set_ylabel('Y Axes')
ax.set_zlabel('Z Axes')
plt.show()
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
▲ 3D绘制的例子
(3) Ex3
import matplotlib as mpl
from mpl_toolkits.mplot3d import Axes3D
import numpy as np
import matplotlib.pyplot as plt
mpl.rcParams['legend.fontsize'] = 10
fig = plt.figure()
ax = fig.gca(projection='3d')
theta = np.linspace(-4 * np.pi, 4 * np.pi, 100)
z = np.linspace(-2, 2, 100)
r = z**2 + 1
x = r * np.sin(theta)
y = r * np.cos(theta)
ax.plot(x, y, z, label='parametric curve')
ax.legend()
plt.show()
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
➤02 绘制Scatter
利用和上面的相同的绘制命令,将原来的plot3D修改成为 scatter即可。
from mpl_toolkits.mplot3d import axes3d
ax = plt.axes(projection='3d')
angle = linspace(0, 2*pi*5, 40)
x = cos(angle)
y = sin(angle)
z = linspace(0, 5, 40)
ax.scatter(x,y,z, color='b')
ax.set_xlabel('X Axes')
ax.set_ylabel('Y Axes')
ax.set_zlabel('Z Axes')
plt.show()
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
▲ Scatter 的例子
➤03 绘制3D Surface
(1) Ex1
▲ 3D surface例子
#!/usr/local/bin/python
# -*- coding: gbk -*-
#============================================================
# TEST2.PY -- by Dr. ZhuoQing 2020-11-16
#
# Note:
#============================================================
from headm import *
from mpl_toolkits.mplot3d import axes3d
ax = plt.axes(projection='3d')
x = arange(-5, 5, 0.1)
y = arange(-5, 5, 0.1)
x,y = meshgrid(x, y)
R = sqrt(x**2+y**2)
z = sin(R)
ax.plot_surface(x, y, z)
ax.set_xlabel('X Axes')
ax.set_ylabel('Y Axes')
ax.set_zlabel('Z Axes')
plt.show()
#------------------------------------------------------------
# END OF FILE : TEST2.PY
#============================================================
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
▲ 3D 绘制Surface
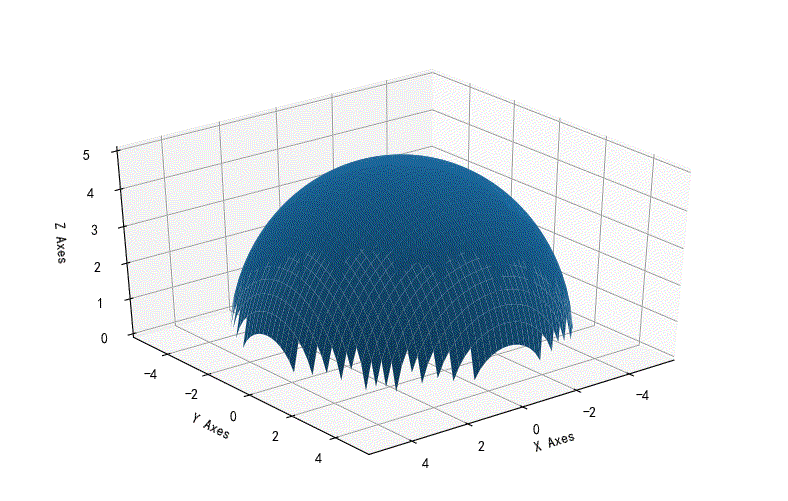
▲ 绘制3D球表面
(2) 举例
'''
======================
3D surface (color map)
======================
Demonstrates plotting a 3D surface colored with the coolwarm color map.
The surface is made opaque by using antialiased=False.
Also demonstrates using the LinearLocator and custom formatting for the
z axis tick labels.
'''
from mpl_toolkits.mplot3d import Axes3D
import matplotlib.pyplot as plt
from matplotlib import cm
from matplotlib.ticker import LinearLocator, FormatStrFormatter
import numpy as np
fig = plt.figure()
ax = fig.gca(projection='3d')
# Make data.
X = np.arange(-5, 5, 0.25)
Y = np.arange(-5, 5, 0.25)
X, Y = np.meshgrid(X, Y)
R = np.sqrt(X**2 + Y**2)
Z = np.sin(R)
# Plot the surface.
surf = ax.plot_surface(X, Y, Z, cmap=cm.coolwarm,
linewidth=0, antialiased=False)
# Customize the z axis.
ax.set_zlim(-1.01, 1.01)
ax.zaxis.set_major_locator(LinearLocator(10))
ax.zaxis.set_major_formatter(FormatStrFormatter('%.02f'))
# Add a color bar which maps values to colors.
fig.colorbar(surf, shrink=0.5, aspect=5)
plt.show()
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
- 32
- 33
- 34
- 35
- 36
- 37
- 38
- 39
- 40
- 41
▲ 彩色表面绘制
➤04 绘制contour
#!/usr/local/bin/python
# -*- coding: gbk -*-
#============================================================
# TESTCONTOUR.PY -- by Dr. ZhuoQing 2020-11-16
#
# Note:
#============================================================
from headm import *
delta = 0.025
x = arange(-3.0, 3.0, delta)
y = arange(-2.0, 2.0, delta)
X,Y = meshgrid(x,y)
Z1 = exp(-X**2-Y**2)
X2 = exp(-(X-1)**2-(Y-1)**2)
Z=(Z1-X2)*2
CS=plt.contour(X,Y,Z)
plt.xlabel("X")
plt.ylabel("Y")
plt.grid(True)
plt.clabel(CS, inline=1, fontsize=8)
plt.title("Simplest default with labels")
plt.tight_layout()
plt.show()
#------------------------------------------------------------
# END OF FILE : TESTCONTOUR.PY
#============================================================
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
1.Ex1
z ( x , y ) = e − x 2 − y 2 − e − ( x − 1 ) 2 − ( y − 1 ) 2 z\left( {x,y} \right) = e^{ - x^2 - y^2 } - e^{ - \left( {x - 1} \right)^2 - \left( {y - 1} \right)^2 } z(x,y)=e−x2−y2−e−(x−1)2−(y−1)2
▲ 绘制的contour例子
2.Ex2
delta = 0.025
x = arange(-3.0, 3.0, delta)
y = arange(-2.0, 2.0, delta)
X,Y = meshgrid(x,y)
Z1 = exp(-(X+1)**2-(Y+0.5)**2)
Z2 = exp(-(X-1)**2-(Y-0.5)**2)
Z=(Z1-Z2)*2
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
Z ( x , y ) = e − ( x + 1 ) 2 − ( y + 0.5 ) 2 − e − ( x − 1 ) 2 − ( y − 0.5 ) 2 Z\left( {x,y} \right) = e^{ - \left( {x + 1} \right)^2 - \left( {y + 0.5} \right)^2 } - e^{ - \left( {x - 1} \right)^2 - \left( {y - 0.5} \right)^2 } Z(x,y)=e−(x+1)2−(y+0.5)2−e−(x−1)2−(y−0.5)2
▲ 函数轮廓
from mpl_toolkits.mplot3d import axes3d
from matplotlib import cm
ax = plt.axes(projection='3d')
ax.set_xlabel('X Axes')
ax.set_ylabel('Y Axes')
ax.set_zlabel('Z Axes')
delta = 0.025
x = arange(-3.0, 3.0, delta)
y = arange(-2.0, 2.0, delta)
X,Y = meshgrid(x,y)
Z1 = exp(-(X+1)**2-(Y+0.5)**2)
Z2 = exp(-(X-1)**2-(Y-0.5)**2)
Z=(Z1-Z2)*2
#CS=plt.plot_surface(X,Y,Z, camp=cm.coolwarm)
ax.plot_surface(X,Y,Z, cmap=cm.coolwarm)
plt.show()
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
文章来源: zhuoqing.blog.csdn.net,作者:卓晴,版权归原作者所有,如需转载,请联系作者。
原文链接:zhuoqing.blog.csdn.net/article/details/109731236
- 点赞
- 收藏
- 关注作者
评论(0)